To generate the RANDC values using the RAND variable in the System Verilog. We have to take one queue and write the constraint on the queue values with unique constructs.
Then the randomly generated values should be assigned to the queue using the push_back method in the post_randomize method.
This is the common question asked by the interviewer to design verification engineers. We have put the code so you can run and understand it well.
//This code is helpful to generate randc value of variable using rand operator.
class packet;
rand bit[3:0]addr;
bit[3:0] my_que[$]; //defining the queue which can store the values of variable
constraint add_cons {
addr inside {0,1,2,3,4,5,6,7}; //value should be randomize inside 0 to 7
unique{addr,my_que}; //consider unique value
};
//pre_randomize method
function void pre_randomize();
if(my_que.size()==8) my_que={}; //assign the size
endfunction
//post_randomize method
function void post_randomize();
my_que.push_back(addr);
endfunction
endclass
module top;
initial begin
packet p;
p=new();
repeat(8) begin
p.randomize(); //randomization method to randomize variables
$display("value of addr=%0d", p.addr);
end
end
endmodule
Simulation Result:
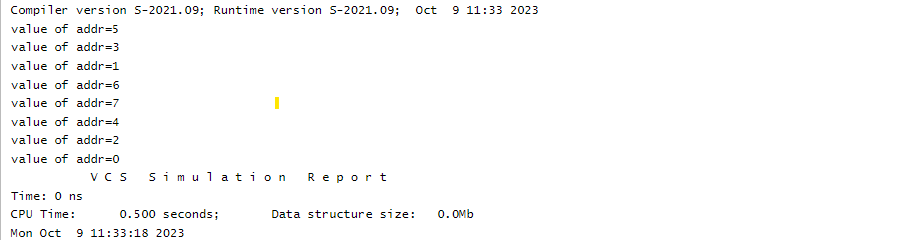